> |
#Definition of a Procedure in Maple:
hello := proc() |
 |
(1) |
 |
(2) |
> |
local a; #a will be a local variable inside the procedure |
 |
(3) |
 |
(4) |
Error, invalid input: f uses a 1st argument, x, which is missing
|
|
> |
#Types in Maple:
whattype(a); |
 |
(5) |
> |
whattype(2);
g:= x->x^2;
whattype(g); |
 |
(6) |
 |
(6) |
 |
(6) |
 |
(7) |
 |
(8) |
 |
(9) |
 |
(10) |
 |
(11) |
 |
(12) |
> |
s:="sin(2.1)+cos(5.1)"; |
 |
(13) |
 |
(14) |
 |
(15) |
 |
(16) |
 |
(17) |
 |
(18) |
 |
(19) |
 |
(20) |
> |
whattype(1..10);
myset:=[seq(1..5,0.5)]; |
 |
(21) |
![`:=`(myset, [1, 1.5, 2.0, 2.5, 3.0, 3.5, 4.0, 4.5, 5.0])](maple-images2/Maple_Programming_Intro_24.gif) |
(21) |
> |
whattype(myset);
nops(myset); #nops returns the number of operands, in this case operands are members of the list
e:= a > b; |
 |
(22) |
 |
(22) |
 |
(22) |
 |
(23) |
 |
(24) |
 |
(25) |
 |
(26) |
 |
(27) |
 |
(28) |
> |
op(f); #finds the operant of a expression |
 |
(29) |
> |
op(0,f); #finds the operator of an expression |
 |
(30) |
 |
(31) |
 |
(32) |
 |
(33) |
 |
(34) |
 |
(35) |
 |
(36) |
 |
(37) |
 |
(38) |
 |
(39) |
 |
(40) |
Error, (in unassign) cannot unassign `x+y' (argument must be assignable)
|
|
 |
(41) |
> |
my_fact := proc(x::integer) |
> |
local i; # 'i' will be a local variable |
> |
local result;
if x > 0 then |
> |
error "Invalid argument"; |
 |
(43) |
Error, invalid input: my_fact expects its 1st argument, x, to be of type integer, but received 2.1
|
|
Error, (in my_fact) Invalid argument
|
|
> |
fib := proc(x::integer) |
> |
b := 3;
print(a);
print(b);
c := 5; |
> |
c := a + b;
if ( c <= x) then |
> |
print(c);
else
break;
end if; |
 |
(45) |
 |
(45) |
 |
(45) |
 |
(45) |
 |
(45) |
 |
(45) |
 |
(45) |
 |
(45) |
 |
(45) |
> |
#Note that reason we still see 89 is that maple returns the last calculation done in the proc as well |
> |
local i,m :: numerics;
description "finds the maximum of a list of numbers"; |
> |
if nargs=0 then #nargs is the number of arguments |
> |
m:= args[1]; #arguments can be grabbed from args[i] list |
> |
for i from 2 to nargs do |
> |
return m; #explicit return, otherwise maple's procedure will return the value of last statement
end if; |
 |
(47) |
 |
(48) |
 |
(49) |
Error, (in my_MAX) cannot determine if this expression is true or false: 2 < s
|
|
> |
save my_MAX, "My_Procs.mpl"; #Saves the procedure in a text file |
> |
#Procedure to integrate a given function numerically: |
> |
my_int:=proc(f::operator, N:: integer, xmin::float, xmax::float) |
> |
local x;
local dx;
dx := (xmax-xmin)/(N-1); |
> |
x:=Array([seq(xmin+i*dx,i=0..N-1)]); |
> |
intf:=Array(1..N);
intf[1]:=0; |
> |
intf(i) := intf(i-1) + ( f(x[i])+f(x[i-1]) ) / 2 * dx; |
 |
(53) |
> |
intsin:=my_int(h,10000,0.0,evalf(4*Pi,10)); |
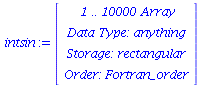 |
(54) |
> |
plot([seq(i*(4*evalf(Pi,10))/9999,i=0..9999)],intsin); |
> |
s:=readline("/home/arman/examples/maple/data.txt"); |
 |
(55) |
> |
a:=readstat("Enter an integer number:"); |
Enter an integer number: |
5: |
 |
(56) |
> |
A:=readdata("data.txt",float);
#Reads the first column |
![`:=`(A, [1.0, 2.0, 3.0])](maple-images2/Maple_Programming_Intro_123.gif) |
(57) |
> |
B:=readdata("data.txt",[float,integer]);
#Reads the first two column |
![`:=`(B, [[1.0, 2], [2.0, 5], [3.0, 4]])](maple-images2/Maple_Programming_Intro_124.gif) |
(58) |
> |
C:=readdata("data.txt",[float,integer,float]); |
![`:=`(C, [[1.0, 2, 3.100], [2.0, 5, 4.200], [3.0, 4, 7.120]])](maple-images2/Maple_Programming_Intro_125.gif) |
(59) |
> |
writedata("data2.txt",C,float); |
last update: Wed May 11, 2016